Isode Support
Guides & Downloads
Find any Administration Guides and API Documentation as well as Deployment Notes for isode products
Isode Support
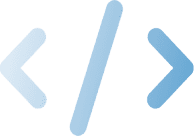
Software Support
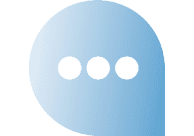
Contact us
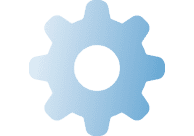
Service Desk
Still need help?
Frequently Asked Questions
If you can’t find what you’re looking for check out our FAQ page, which covers topics on our Products, Support, Licencing and more.